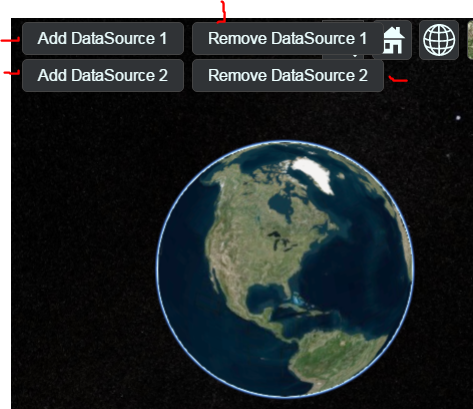
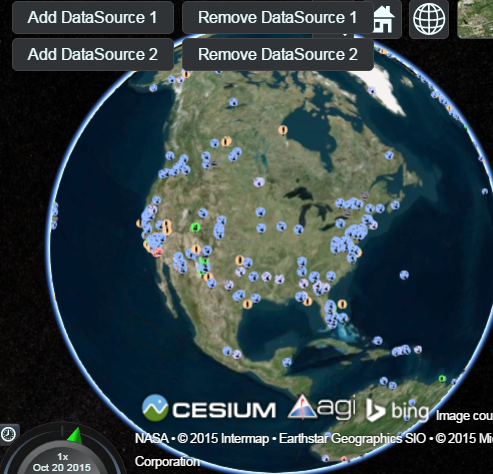
var viewer = new Cesium.Viewer('cesiumContainer');
var dataSource1 = new Cesium.KmlDataSource();
dataSource1.load('../../SampleData/kml/facilities/facilities.kml');
var dataSource2 = new Cesium.KmlDataSource();
dataSource2.load('../../SampleData/kml/gdpPerCapita2008.kmz');
Sandcastle.addToolbarButton('Add DataSource 1', function() {
viewer.dataSources.add(dataSource1);
});
Sandcastle.addToolbarButton('Remove DataSource 1', function() {
viewer.dataSources.remove(dataSource1);
});
Sandcastle.addToolbarButton('Add DataSource 2', function() {
viewer.dataSources.add(dataSource2);
});
Sandcastle.addToolbarButton('Remove DataSource 2', function() {
viewer.dataSources.remove(dataSource2);
});
If you are not familiat with Promises, I recommend reading this article: http://www.html5rocks.com/en/tutorials/es6/promises/ Cesium specifically uses the `when` promise library that is mentioned in the article.
var viewer = new Cesium.Viewer('cesiumContainer');
var promise1 = Cesium.KmlDataSource.load('../../SampleData/kml/facilities/facilities.kml');
var promise2 = Cesium.KmlDataSource.load('../../SampleData/kml/gdpPerCapita2008.kmz');
Cesium.when(promise1, function(dataSource1){
Sandcastle.addToolbarButton('Add DataSource 1', function() {
viewer.dataSources.add(dataSource1);
});
Sandcastle.addToolbarButton('Remove DataSource 1', function() {
viewer.dataSources.remove(dataSource1);
});
});
Cesium.when(promise2, function(dataSource2){
Sandcastle.addToolbarButton('Add DataSource 2', function() {
viewer.dataSources.add(dataSource2);
});
Sandcastle.addToolbarButton('Remove DataSource 2', function() {
viewer.dataSources.remove(dataSource2);
});
});