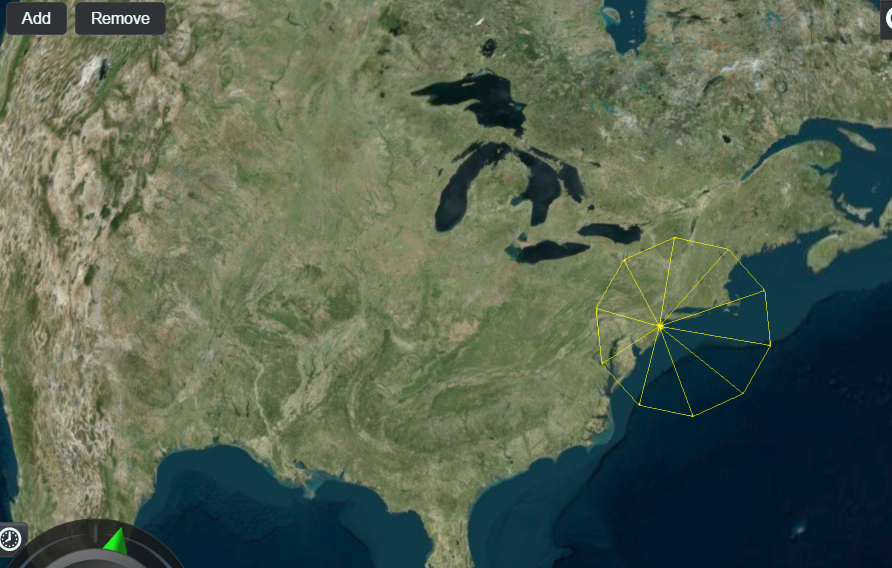
var viewer = new Cesium.Viewer('cesiumContainer');
var scene = viewer.scene;
var ellipsoid = scene.globe.ellipsoid;
var input = {
id: 'ID1',
length: 500000,
topRadius: 300000,
bottomRadius: 1000
};
var myPrimitives = [];
var instance = new Cesium.GeometryInstance({
geometry: new Cesium.CylinderOutlineGeometry({
length: input.length,
topRadius: input.topRadius,
bottomRadius: input.bottomRadius,
slices: 10
}),
modelMatrix: Cesium.Matrix4.multiplyByTranslation(Cesium.Transforms.eastNorthUpToFixedFrame(
Cesium.Cartesian3.fromDegrees(-75.59777, 40.03883)), new Cesium.Cartesian3(0.0, 0.0, 1000000.0), new Cesium.Matrix4()),
id: input.id,
attributes: {
color: Cesium.ColorGeometryInstanceAttribute.fromColor(Cesium.Color.YELLOW)
}
});
function arCylinder(ar){
if (ar === 'a') {
var myPrimitive = new Cesium.Primitive({
geometryInstances: instance,
appearance: new Cesium.PerInstanceColorAppearance({
flat: true
})
});
myPrimitives.push(myPrimitive);
scene.primitives.add(myPrimitive);
}
else if(ar === 'r'){
scene.primitives.remove(myPrimitives.pop());
}
}
Sandcastle.addToolbarButton('Add', function() {
arCylinder('a');
});
Sandcastle.addToolbarButton('Remove', function() {
arCylinder('r');
});